GPaR sofware
Some examples:
The GPM_ConwayGraph is a grid graph: each node of the graph are connected to its 8 neighbors. The edges are oriented from 0 to 7. The coordinates of the node are the index of its row & column. The third coordinate has its value in {UNDEFINED,DEAD,ALIVE,DEAD_OR_ALIVE}
The large graph is a grid of size NxP whose state is predefined.
The pattern graph is a grid 3x3:
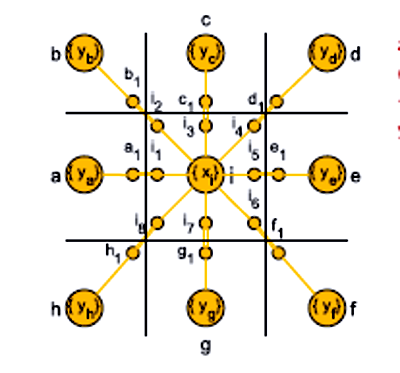
The transform graph is the pattern graph.
Only the third coordinate of the nodes change during the iterations. its state is compute with the conway function GPM_ConwayFunction::updateStates() The rule to apply is to change the coordinates of the center node. The state value is defined as follow:
- compute the sum of states of the neighbor node (DEAD:0 ALIVE:1)
- if the sum is equal to 3, the new state is ALIVE
- if the sum is equal to 2 and the old state is ALIVE, the new state is ALIVE
- otherwise the new state is DEAD.
If a state is DEAD_OR_ALIVE, the 2 cases DEAD & ALIVE are considered. After examining all the cases, if all the state values are DEAD, the new state of the center is DEAD, similarly for ALIVE where as if all the state values are differents, the new state value is DEAD_OR_ALIVE.
This class can make a simulation of conway problem has folow: The input graph is an image of size 200x200 whose pixel size is 10x10 with:
- white pixel (#FFFFFF) for UNDEFINED state,
- red pixel (#FF0000) for ALIVE state,
- grey pixel (#E9DED5) for DEAD state,
- blue pixel for (#0000FF) DEAD_OR_ALIVE state.
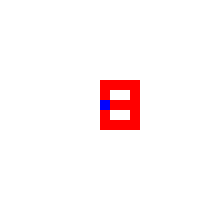
The output graph is an image as follow (click on the image to see the video):
The execution command line is:
gpm_examples.exe –path="output path" –prefix="prefix of the output file name" –imageFile="name of the input file" –iterations="number of iterations to proceed" –inPixelSize=[n,p] –outPixelSize=[n,p] make conway